Hello! So this has been super cool and fun, hope you find it fun too! I’ve been working with JavaScript to make sure we are playing sounds at the exact right times!
Imagine you have two different sound patterns:
- Doom (1-second pause) Doom
- Doom (half a second pause) Doom
To make this work, we need to play these sounds back at just the right moments. We can use a bit of JavaScript magic to play sounds at specific timestamps. Check out this script:
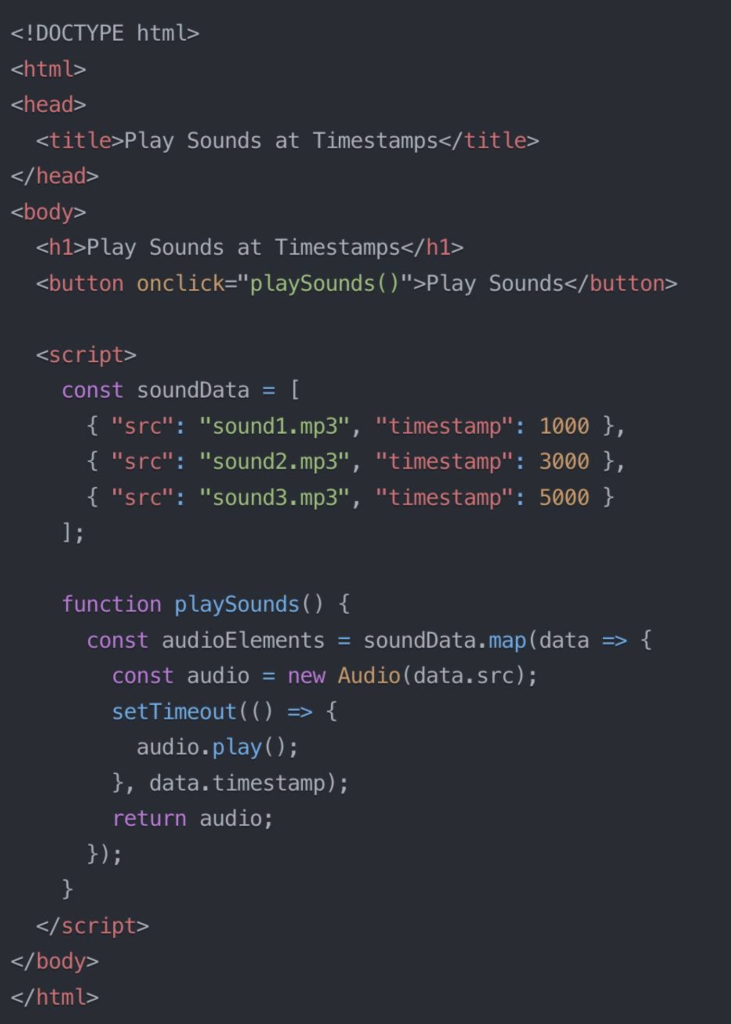
Breaking It Down
- HTML Structure: We have an HTML page with a button that says “Play Sounds.” When you click it, the playSounds() function runs.
- Sound Data: The soundData array lists our sounds (doom and tak real precaution sounds!) with their sources and the times they should play.
- playSounds Function: This function goes through each sound in soundData and uses setTimeout to play each one at the right time.
Our machine learning model first identifies when each sound occurs which we then use to export the timestamps to create our soundData array. Using JavaScript, we can now play the sounds exactly when the AI model hears them! Yay! ???
Here’s an accessible version of the code you can copy and paste if you’d like to give it a go yourself!
Click here if you want to copy and paste the code to try out for yourself! Show code
<!DOCTYPE html>
<html>
<head>
<title>Play Sounds at Timestamps</title>
</head>
<body>
<h1>Play Sounds at Timestamps</h1>
<button onclick="playSounds()">Play Sounds</button>
<script>
const soundData = [
{ "src": "sound1.mp3", "timestamp": 1000 },
{ "src": "sound2.mp3", "timestamp": 3000 },
{ "src": "sound3.mp3", "timestamp": 5000 }
];
function playSounds() {
const audioElements = soundData.map(data => {
const audio = new Audio(data.src);
setTimeout(() => {
audio.play();
}, data.timestamp);
return audio;
});
}
</script>
</body>
</html>